Introduction to the Dart Programming Language - @Dart Basics
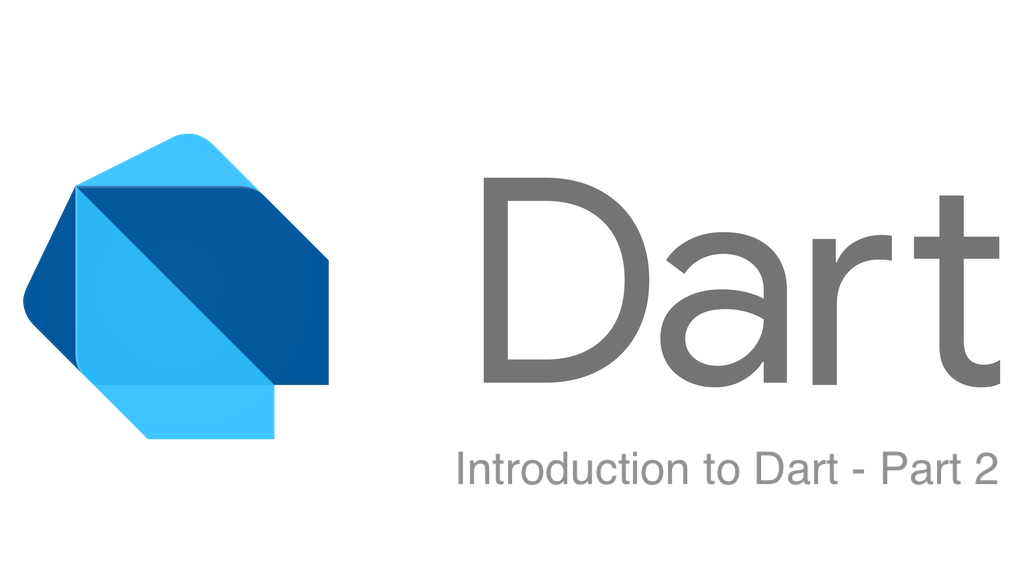
In this article, I will walk through the Dart Basics and start a brief introduction to the dart programming language.
Lets Start with, What exactly is Dart?
Dart is a Google-developed object-oriented programming language. Although it is technically unrestricted, it is typically used to create web front-end user interfaces (using Angular Dart or Flutter for Web) and mobile apps (Flutter). It’s actively developed, compiled to native machine code (when used to construct mobile apps), and highly typed. It’s influenced by contemporary features of other programming languages (namely Java, JavaScript, and C#).
Dart is a compiled language, as previously stated. That is to say, your code is not executed exactly as you wrote it, but rather parsed and transformed by a compiler (to machine code).
Dart is a Statically Typed Programming Language
Dart is a typed programming language, which is not true of all computer languages. But what exactly does that imply?
Every value you utilize in your programme (for example, any user input you’re storing) has a type – for example, “text.” However, in Dart (and pretty much all other programming languages), that would be referred to as “string” rather than “text.” “. There is an exception in Dart about it; that is ‘var’.
If you’re working with a user’s age, on the other hand, you may be utilizing a number with no decimal places – a “integer” value. “Doubles” are numbers having decimal places (or “float” – in other programming languages).
1. | string | String myName = ‘John’; | Text is held in this container. You can use single or double quotes; just stick to it once you’ve decided. |
2. | num, int, double | int age = 25; double price = 5.6 | In Dart, there are two sorts of numbers: integers (numbers without a decimal place) and doubles (numbers with a decimal place) (numbers with decimal places) |
3. | object | Person = Person() | In Dart, everything is an object, even integers. However, as seen below, objects can be more complicated. |
Variables
You’ll almost always need to save certain values in your applications. In memory, not necessarily in a database or a file. You could need to save an interim result, a user’s input before processing it, or information about your Flutter widget (for example, “Should it be displayed on the screen right now?”).
Variables are used to store such information (= values).
Variables are data containers that have names and can hold any sort of value.
For example:
int is a type variable that holds a 25-bit integer value.
var myAge; myAge = 20;
Dart knows that myAge is a variable because of the var keyword. In addition to notifying Dart about the variable, you may also “inform Dart” about the type of data stored in the variable by using the type name instead of var. e.g.
int myAge; myAge = 20;
Dart, on the other hand, contains a feature called “type inference”
Dart is quite good at inferring kinds of values, as seen by this. Because you initialize the variable (that is, you assign a value straight away) with an integer value but using var keyword, Dart may deduce that myAge is of type int even though you created it with var.
Because of the built-in type inference, it’s regarded best practice to use var instead of explicitly declaring the type when defining variables. As a result, this excerpt is preferred:
var myAge = 25;
Such changes if you create a variable without an initial value; in that case, you must tell Dart what kind of data you intend to save in it:
int myAge; myAge = 25;
Functions
Aside from variables, “functions” are another important component of every programming language. You may “outsource” code into “re-usable code snippets” using functions. Here’s an illustration:
var price1 = 9.99; var price2 = 10.99; var total = price1 + price2; var numOfRounds1 = 10; var numOfRounds2 = 45; var totalRounds = numOfRounds1 + numOfRounds2;
We have the identical logic of adding two integers in two distinct locations in our code in this example. Rather of repeating ourselves, it would be wonderful if we could put that reasoning into a function that we could call whenever we wanted. Here’s the updated snippet, which makes use of a function:
num addNumbers(num n1, num n2) { return n1 + n2; } var price1 = 9.99; var price2 = 10.99; var total = addNumbers(price1, price2); var numOfRounds1 = 10; var numOfRounds2 = 45; var totalRounds = addNumbers(numOfRounds1, numOfRounds2);
Of course, at first glance, this code appears to have become longer and more complicated. However, the benefit is that if you ever need to alter your addition logic, you can do so in one place => within the function.
To alter a + to a -, for example, you don’t have to update numerous locations in code (if you wanted to do that).
Arguments (the data between the ()) are essentially variables that are scoped to the function. The term “scoping” refers to the fact that the variables can only be used within the block statement (= function body, the code between the ) where they were declared.
Furthermore, functions can return values, such as the result of this example’s addition.
What does it mean to be “object-oriented”?
Dart is an object-oriented programming language, which implies that each value is an object in Dart. Even a single digit.
But what exactly are “objects”?
Objects are data structures that may be found in many different computer languages. Even primitive items like text (= String) or integers (= Integers and Doubles) are objects in Dart. However, you may create your own objects or use more sophisticated built-in objects (such as lists of data).
If you wish to describe more complicated data relationships or encapsulate specific functionality in “one building block,” you’ll frequently create your own objects.
Hope you enjoyed it, I focused on object’s usage and other object oriented concepts in my next tutorial here.