Writing Kubernetes CronJobs: A Simple Guide
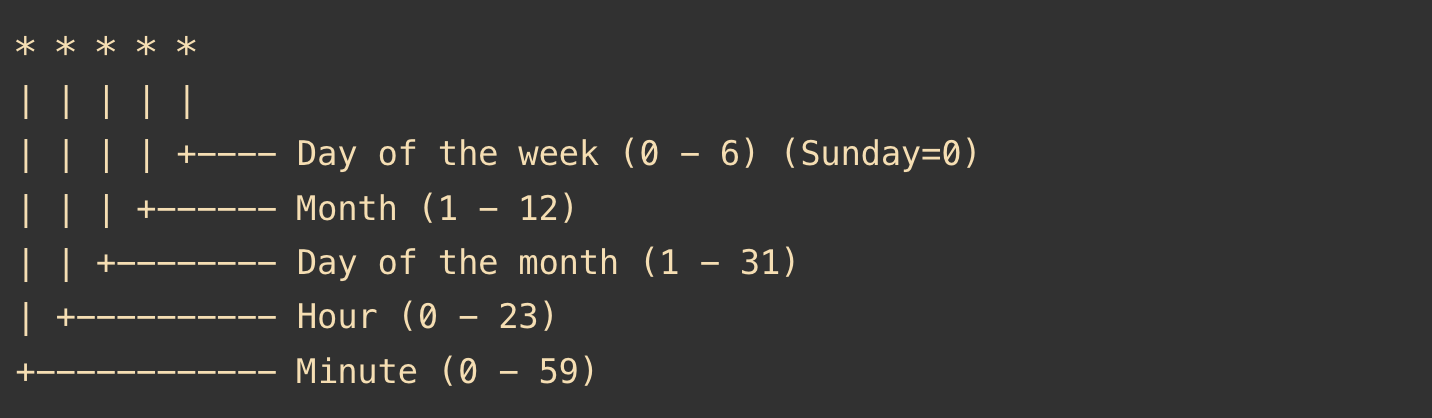
Kubernetes CronJobs are a powerful tool for running scheduled tasks within your Kubernetes cluster. Think of them as cron jobs for containers — they allow you to automate tasks like backups, batch jobs, or routine cleanups. In this guide, we'll walk through how to set up a CronJob in Kubernetes with a simple example.
What is a Kubernetes CronJob?
A CronJob in Kubernetes is essentially a job that runs on a scheduled basis. It allows you to run containers periodically, much like the cron utility on Linux, but with the added benefits of Kubernetes' orchestration.
When to Use Kubernetes CronJobs
CronJobs are ideal for tasks that need to run at specific intervals. For instance, you might use a CronJob for:
- Database backups that run every night.
- Sending email reports once a day.
- System cleanups that run weekly.
Setting Up a Kubernetes CronJob
To create a CronJob, you’ll define it in a YAML file, specifying the schedule and the task you want it to run. Here’s a basic example:
apiVersion: batch/v1
kind: CronJob
metadata:
name: daily-backup
spec:
schedule: "0 2 * * *" # Run at 2 AM every day
jobTemplate:
spec:
template:
spec:
containers:
- name: backup-container
image: my-backup-image:latest
args:
- /bin/sh
- -c
- "tar -czf /backup/daily-backup.tar.gz /data"
restartPolicy: OnFailure
Breaking Down Kubernetes CronJob
- apiVersion: Defines the version of the Kubernetes API you're using.
- kind: Specifies that we're creating a CronJob.
- schedule: This is the cron syntax to set when the job runs. In this case, it’s set to run daily at 2:00 AM.
- jobTemplate: Defines the job itself. This includes:
- container name: The name of the container you want to run.
- image: The Docker image used for the container.
- args: The commands executed by the container (in this case, a backup command).
Testing Your Kubernetes CronJob
Once you’ve applied the CronJob, you can check its status by running:
kubectl get cronjobs
To view the logs for a specific CronJob execution, use:
kubectl logs <pod-name>
If you need to troubleshoot, you can also describe the CronJob:
kubectl describe cronjob daily-backup
Managing Kubernetes CronJob Frequency
CronJob schedules follow the cron syntax, which looks like this:
* * * * *
| | | | |
| | | | +---- Day of the week (0 - 6) (Sunday=0)
| | | +------ Month (1 - 12)
| | +-------- Day of the month (1 - 31)
| +---------- Hour (0 - 23)
+------------ Minute (0 - 59)
For example:
"0 3 * * *"
runs the job at 3 AM every day."*/15 * * * *"
runs the job every 15 minutes.
Tip About Kubernetes Crons:
Make sure to also specify the startingDeadlineSeconds
field to control how long the CronJob will wait for the job to complete before considering it failed. Avoid a Forever running job 😄