Simple Python Script to Tell Jokes - Cool Scripts 1
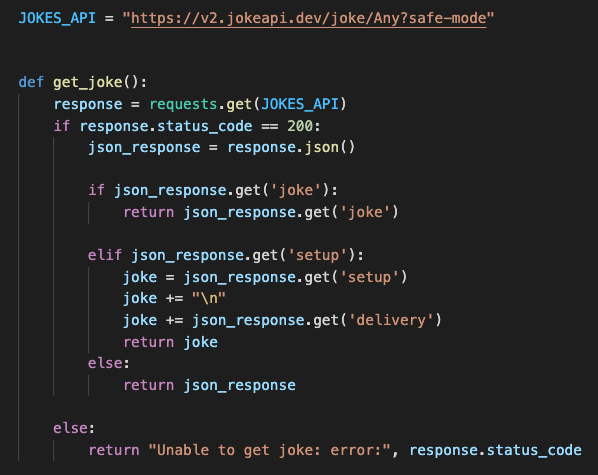
This cool python script to tell jokes is based on the Jokes API. This is a one of the coolest REST API that I found online.
To write a python script that can make use of this Jokes API, you would need the following:
- Python
- Any Code Editor
- Internet
The following is the full code for this script written in python3
# This program will use the following API # https://v2.jokeapi.dev/joke/Any?safe-mode # If above API is unreachable, this script will not work #### instructions to run #### ## save this file as jokes.py ## ## pip install requests ## ## python jokes.py ## import requests JOKES_API = "https://v2.jokeapi.dev/joke/Any?safe-mode" def get_joke(): response = requests.get(JOKES_API) if response.status_code == 200: json_response = response.json() if json_response.get('joke'): return json_response.get('joke') elif json_response.get('setup'): joke = json_response.get('setup') joke += "\n" joke += json_response.get('delivery') return joke else: return json_response else: return"Unable to get joke: error:", response.status_code if __name__ == "__main__": print(get_joke())
You could download this script file jokes.py
How to Run This Script
This script has one dependency, that is requests. Before running this, the requests module is needed to install and could be installed using pip. Run the following two commands in command prompt or terminal from the directory where you save this script.
pip install requests python jokes.py or python3 jokes.py
All credit goes to the author of this API: Author of Jokes API