Port scanning using esp32 and micropython
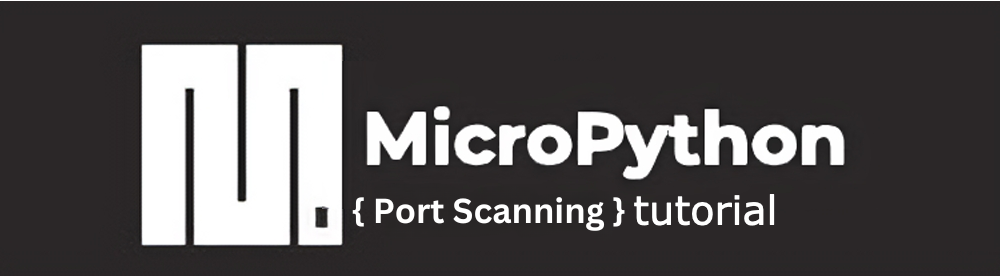
In this tutorial we will be coding our own port scanner for port scanning using esp32 and micropython. Please note that this is for educational purposes only. Scanning networks without permission can be illegal and unethical. Lets dive into a brief introduction of port scanning, why its unethical and then coding one for us.
What is Port Scanning?
Port scanning is a way to discover open spots, called ports, on a computer's network. This helps to understand which parts of the network or computer on network can talk to the outside world.
Why Port Scanning is Unethical?
Scanning ports on someone else's network is not only unethical but also illegal in many countries. Its similar to the methodically checking all doors and windows in a building to find which ones are open. Hackers usually use this method on a network or computer to see how far they can reach and what they can access on target computer or network.
Other uses of Port Scanning
You might have question, if its unethical why the hell this information is available so easily and people like me are giving away the code to perform this scan. The one major use of port scanning is in vulnerability scanning. To perform an assessment about how vulnerable a network is to cyber attacks or security breaches.
Code to Connect to The Network for Performing Scan
import network
import time
# Initialize Wi-Fi Interface
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
# wifi creds
ssid = '<YOUR WIFI SSID>'
password = '<YOU WIFI PASSWORD>'
print(wlan.isconnected())
time.sleep(0.5)
# Connect to the Wi-Fi network
wlan.connect(ssid, password)
print('Connected to Wi-Fi')
Complete Code for Port Scanner using Micropython
import socket
import time
import network
# Initialize Wi-Fi Interface
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
# wifi creds
ssid = '<YOUR WIFI SSID>'
password = '<YOU WIFI PASSWORD>'
print(wlan.isconnected())
time.sleep(0.5)
# Connect to the Wi-Fi network
wlan.connect(ssid, password)
print('Connected to Wi-Fi')
###
print(wlan.ifconfig())
###
def scan_port(ip, port):
s = usocket.socket(usocket.AF_INET,usocket.SOCK_STREAM)
try:
s.settimeout(5)
s.connect((ip, port))
return True
except OSError as e:
# print(e) ## uncomment for debugging
return False
finally:
s.close()
def port_scanner(ip, start_port, end_port):
print("Scanning IP: ", ip)
for port in range(start_port, end_port+1):
if scan_port(ip, port):
print("Port", port, "is open")
else:
print("Port", port, "is closed")
# Example usage
ip_to_scan = '198.1.0.1' # Replace with the IP you want to scan
start_port = 1
end_port = 100 # Scanning 100 ports, adjust as needed
## starting port scaning
print("Running port scaning..")
port_scanner(ip_to_scan, start_port, end_port)
main.py
In above code, replace the value of ip_to_scan with the IP where you want to perform test and provide the range of ports in start_port and end_port. Save the code as main.py on you micropython enabled device.
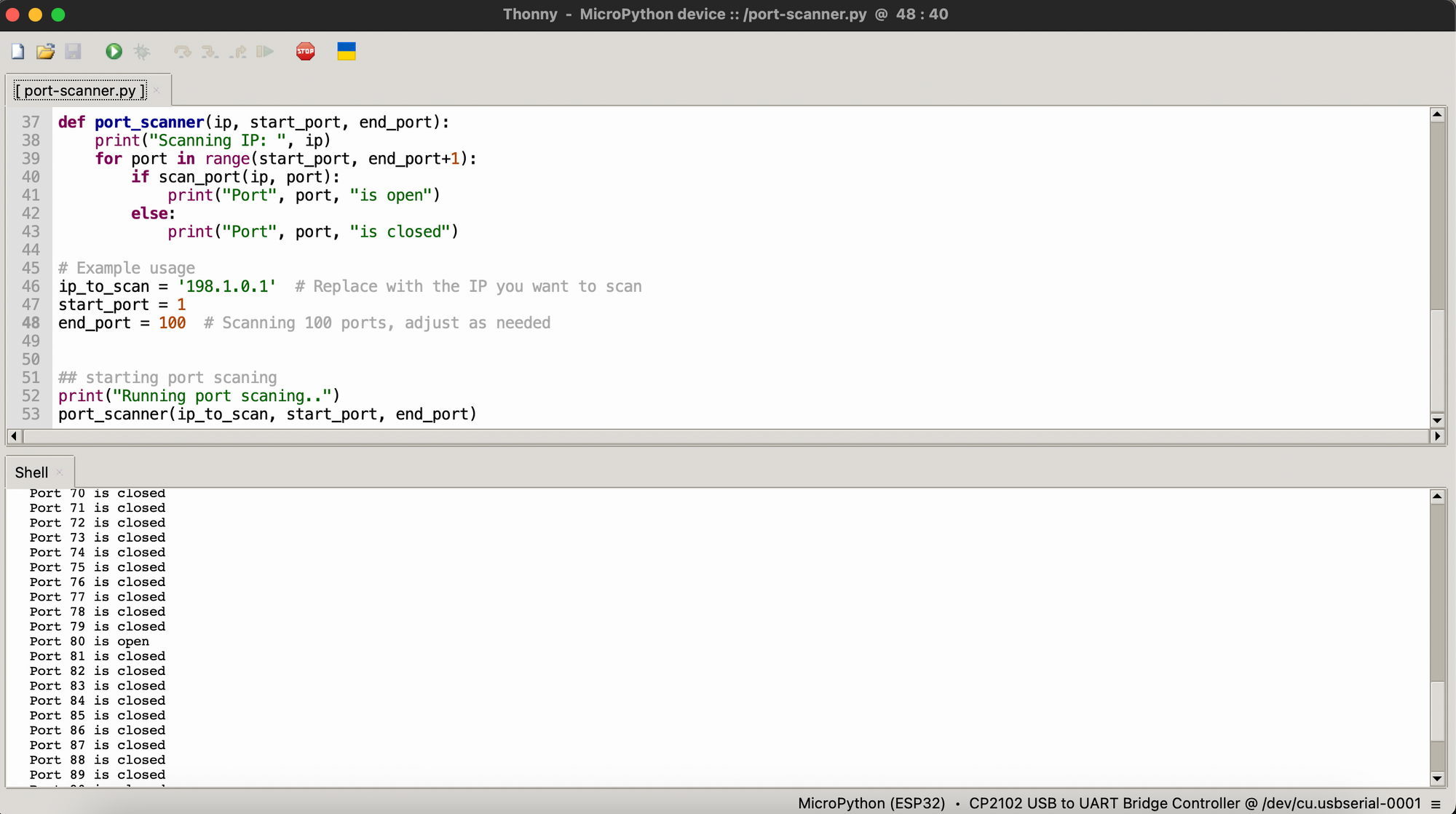
Voila! you get your esp32 ready for port scanning !