Multiple http requests with python- Fastest way
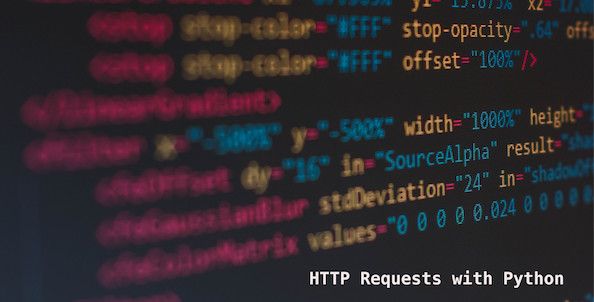
There are many different methods to send multiple HTTP requests with python. The most common methods are, using multi-threading, sessions framework, tornado and aiohttp package.
In this tutorial, I am going to make a request client with aiohttp package and python 3.
The aiohttp package is one of the fastest package in python to send http requests asynchronously from python. Support post, json, REST APIs.
Steps to send asynchronous http requests with aiohttp python
Step1 :
Install aiohttp
pip install aiohttp[speedups]
Step2:
Code to send asynchronous http requests with aiohttp
import aiohttp import asyncio async def get(url): async with aiohttp.ClientSesion() as session: async with session.get(url) return response loop = asyncio.get_event_loop() multiple_requests = [get("http://your-website.com") for _ in range(10)] results = loop.run_until_complete(asyncio.gather(*multiple_requests)) print("Results: %s" % results)
Step3:
change http://your-website.com to the url on which you want to send requests
Save above code as multiple-requests.py
and run it with following command:
python3 multiple-requests.py
Congrats !! you can now send multiple http requests asynchronously by using python.
JSON Add on: With the help of above scrip you can send a get request to a URL, if you want to send JSON data to a REST client with same method, use the following code in Step2:
import aiohttp import asyncio async def get(url): async with aiohttp.ClientSesion() as session: async with session.post(url, json={'name':'test'}) return response loop = asyncio.get_event_loop() multiple_requests = [get("http://your-website.com") for _ in range(10)] results = loop.run_until_complete(asyncio.gather(*multiple_requests)) print("Results: %s" % results)