How to send email using esp32 with micropython and brevo
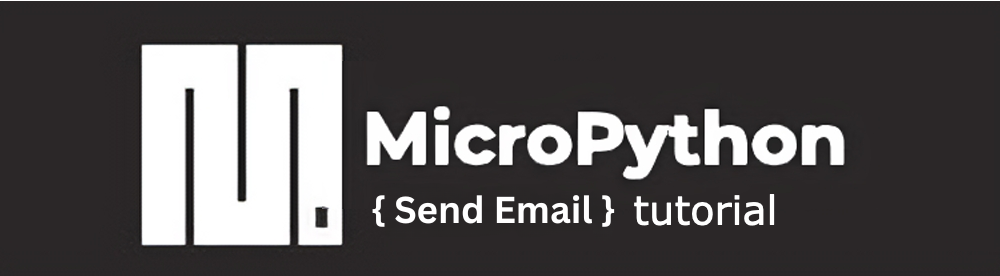
Brevo (formally Sendinblue) is widely used for its reliability and ease of integration, offers a REST API for sending emails. In this tutorial we will go step by step about how to send email using esp32 with micropython and brevo
Prerequisites
- ESP32 Module: With MicroPython firmware installed.
- Brevo Account: Sign up for a free brevo account here.
- Brevo API Key: Create an API key in your Brevo account.
Step-by-Step Guide
Step 1: Setting Up Your Environment
- Connect ESP32 to Your Computer
- Use Thonny to write and save your code
Step 2: Code to Connect ESP32 to Wi-Fi
import network
##### Initialize Wi-Fi Interface
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
#### wifi creds
ssid = '<YOUR-SSID>'
password = '<YOUR-PASSWORD>'
print(wlan.isconnected())
time.sleep(0.5)
# Connect to the Wi-Fi network
wlan.connect(ssid, password)
Step 3: Upload the urequests library to esp32
This library can be single file named urequests.py or a folder named "urequests" with a "__init__.py" file inside. In the code example attached I used the library with folder. You can download the file from here and extract the init file to urequests folder inside root directory of esp32.
It should look like this: /urequests/__init__.py
Step 4: Sending an Email using Brevo API
import urequests
import ujson
brevo_api_key = '<YOUR-API-KEY-HERE>'
url = 'https://api.brevo.com/v3/smtp/email'
headers = {
'accept': 'application/json',
'api-key': brevo_api_key,
'content-type': 'application/json',
}
data = {
"sender": {
"name": "ESP32 CLIENT",
"email": "<YOUR-EMAIL-ID>"
},
"to": [
{
"email": "<RECEIVER-EMAIL-ID>",
"name": "<RECEIVER NAME>"
}
],
"subject": "Hello world from Esp32",
"htmlContent": "<html><head></head><body><p>Hello,</p>This is my first test email sent from esp32 using Brevo.</p></body></html>"
}
response = urequests.post(url, data=ujson.dumps(data), headers=headers)
Complete Code for sending email from esp32
import urequests
import ujson
import network
import time
# Initialize Wi-Fi Interface
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
wlan.disconnect()
# wifi creds
ssid = '<YOUR-SSID>'
password = '<YOUR-PASSWORD>'
print(wlan.isconnected())
time.sleep(0.5)
# Connect to the Wi-Fi network
wlan.connect(ssid, password)
print('Connected to Wi-Fi')
brevo_api_key = '<YOUR-API-KEY-HERE>'
url = 'https://api.brevo.com/v3/smtp/email'
headers = {
'accept': 'application/json',
'api-key': brevo_api_key,
'content-type': 'application/json',
}
data = {
"sender": {
"name": "ESP32 CLIENT",
"email": "<YOUR-EMAIL-ID>"
},
"to": [
{
"email": "<RECEIVER-EMAIL-ID>",
"name": "<RECEIVER NAME>"
}
],
"subject": "Hello world from Esp32",
"htmlContent": "<html><head></head><body><p>Hello,</p>This is my first test email sent from esp32 using Brevo.</p></body></html>"
}
response = urequests.post(url, data=ujson.dumps(data), headers=headers)
if response.status_code == 201:
print("Email sent successfully!")
else:
print("Failed to send email. Response:", response.text)
response.close()
code to send email from esp32
Voila! this is all you need to send email from esp32. You can download this code with requests library in the file below and place on your esp32 (add your api key and emails) in places like <YOUR-API-KEY-HERE>