How to Download Mp3 Files with Python
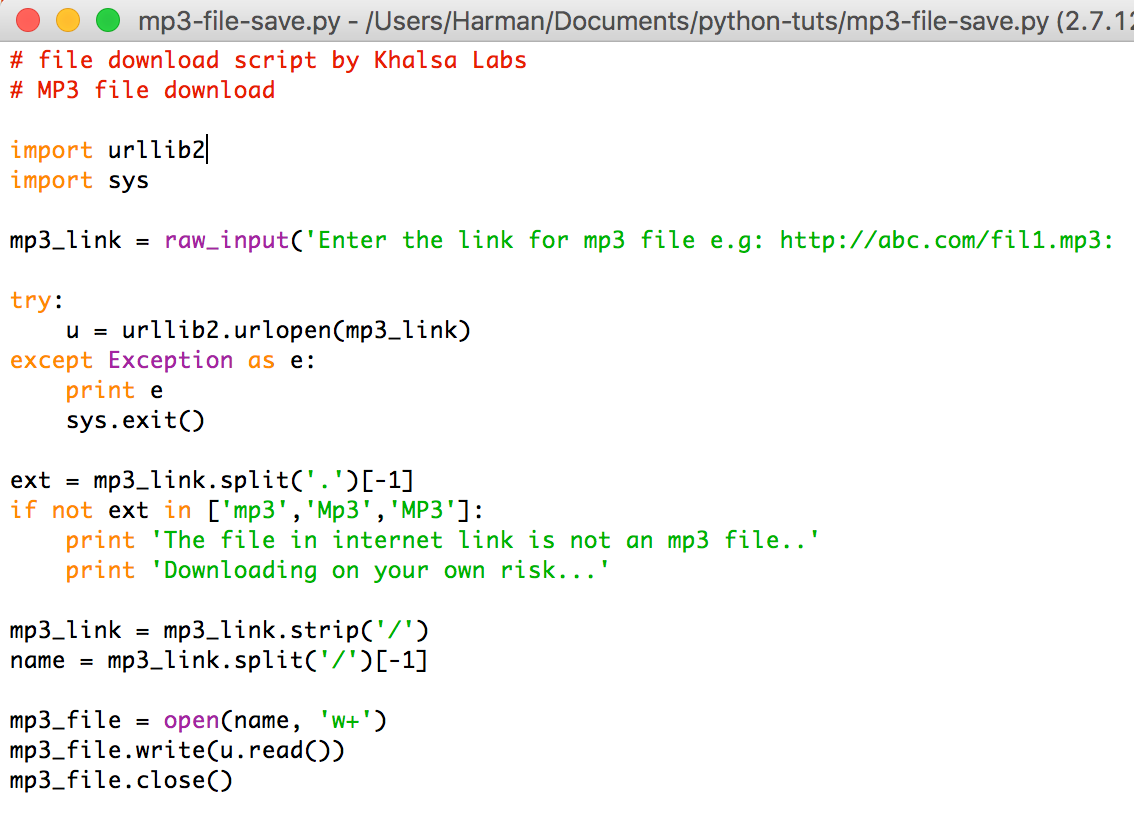
Lets Write a Code to Download Mp3 files from internet in Python using terminal or by executing script.
In this tutorial, I am sharing a script in python with which one can download audio mp3 files from internet through terminal.
To run this script, either copy paste the code below in your python idle and execute it or download and execute the python file from the link at the end of post.
# file download script by Khalsa Labs # MP3 file download import urllib2 import sys mp3_link = raw_input('Enter the link for mp3 file e.g: http://abc.com/fil1.mp3: ') try: u = urllib2.urlopen(mp3_link) except Exception as e: print e sys.exit() ext = mp3_link.split('.')[-1] if not ext in ['mp3','Mp3','MP3']: print 'The file in internet link is not an mp3 file..' print 'Downloading on your own risk...' mp3_link = mp3_link.strip('/') name = mp3_link.split('/')[-1] mp3_file = open(name, 'w+') mp3_file.write(u.read()) mp3_file.close()
If you guys wonder, why I write code to do the function that can be done simply by browser then there are two reasons to answer your curiosity;
This Script can be used to download files through terminal.
This Script can be combined together with other code to write a download manager software.
You can download a zip file containing code to download mp3 file in python, file of above code at mp3-file-save.py.