How Call a REST API from ESP32 Using MicroPython
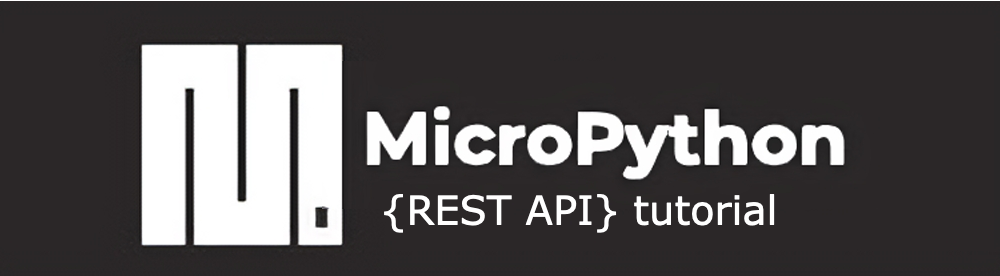
To call a REST API from an ESP32 using MicroPython, you'll typically need to connect the ESP32 to a Wi-Fi network and then use HTTP methods to interact with the API. Below is a step-by-step tutorial to achieve this.
We will use micropython in this tutorial to call REST API from ESP32:
What do you need
- ESP32 with MicroPython Installed: Learn how to install micropython on esp32 here
- Wi-Fi network Details (SSID and password).
Step 1: Code to Connect ESP32 to Wi-Fi
import network
# Initialize Wi-Fi Interface
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
# wifi creds
ssid = 'your_wifi_ssid'
password = 'your_wifi_password'
# Connect to Your Wi-Fi:
while not wlan.isconnected():
pass
print('Connected to Wi-Fi'))
Step 2: Install urequests Library (If Not Present)
The urequests library is used for making HTTP requests. It may or may not be included in your MicroPython firmware (its included in latest micropython).
If you are using older version of micropython, lets download the urequest with this simple step:
Download the file from here and save it on the root directory of your esp32 (using thonny or any file transfer tool like ampy). Using thonny it will be easier.
Step 3: Code to Call REST API
import urequests
# calling a publicaly available REST API
url = 'http://api.example.com/data'
response = urequests.get(url)
# checking the response
if response.status_code == 200:
print(response.text) # printing API response
else:
print('Failed. Error code:', response.status_code)
Step 4: Run Your Code (complete code here)
import network
import urequests
# Initialize Wi-Fi Interface
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
# wifi creds
ssid = 'your_wifi_ssid'
password = 'your_wifi_password'
# Connect to Your Wi-Fi:
while not wlan.isconnected():
pass
print('Connected to Wi-Fi'))
# calling a publicaly available REST API
url = 'https://dog.ceo/api/breeds/list/all' # you can use your API url here
response = urequests.get(url)
# checking the response
if response.status_code == 200:
print(response.text) # printing API response
else:
print('Failed. Error code:', response.status_code)
REST API Call from esp32 using micropython
- save above code file named as main.py and transfer to your esp32 (using thonny). You can also directly save file using thonny to your esp32. (shown in image)
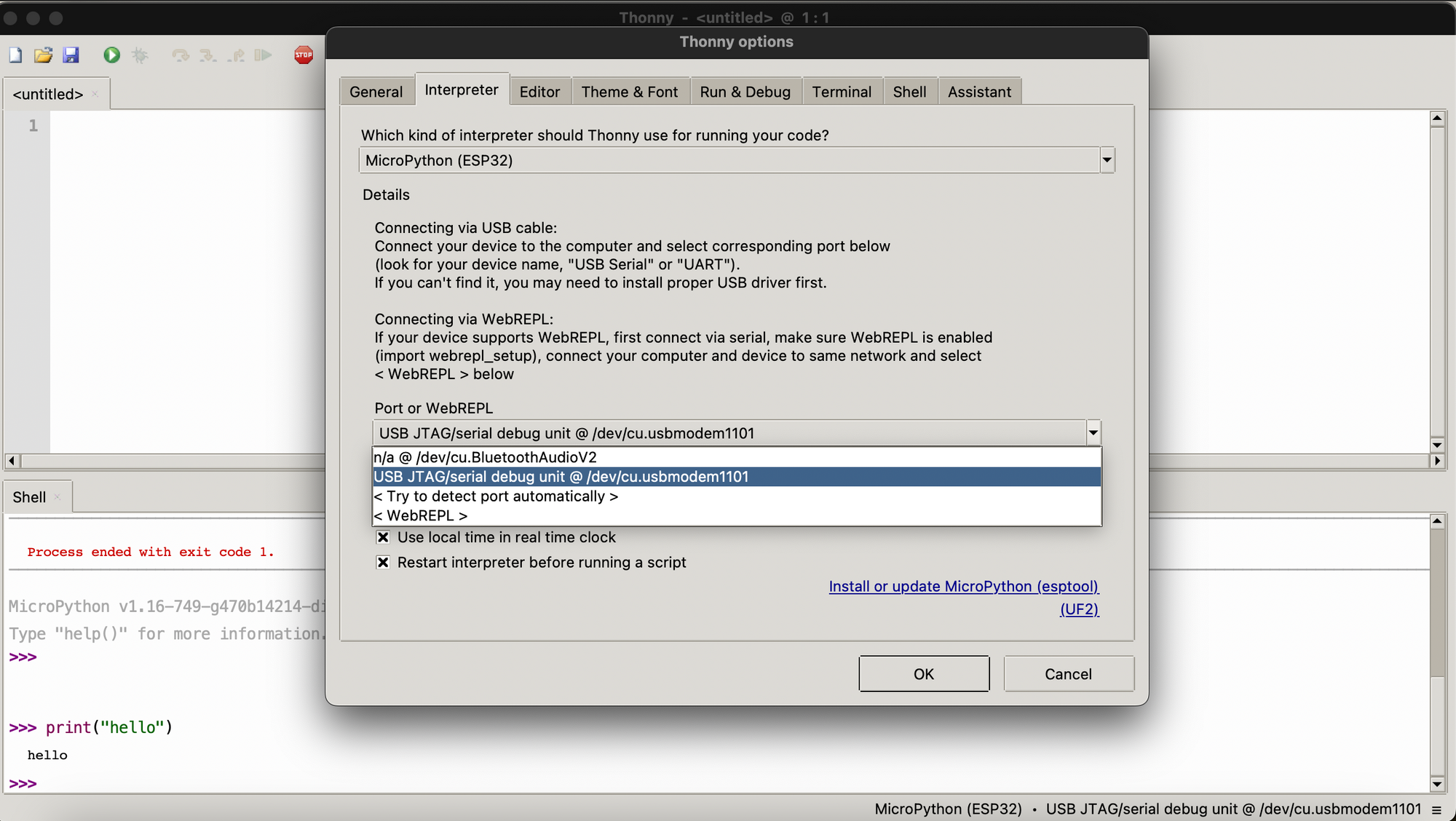
- Reset esp32 and you will see the API call going from esp32, result will be printed on console (thonny' console).
Step 5: Verify the Output
Check the output on the serial console (thonny's serial console). You should see the response from the REST API! Cheers