Hello World in Flutter, and why Flutter?
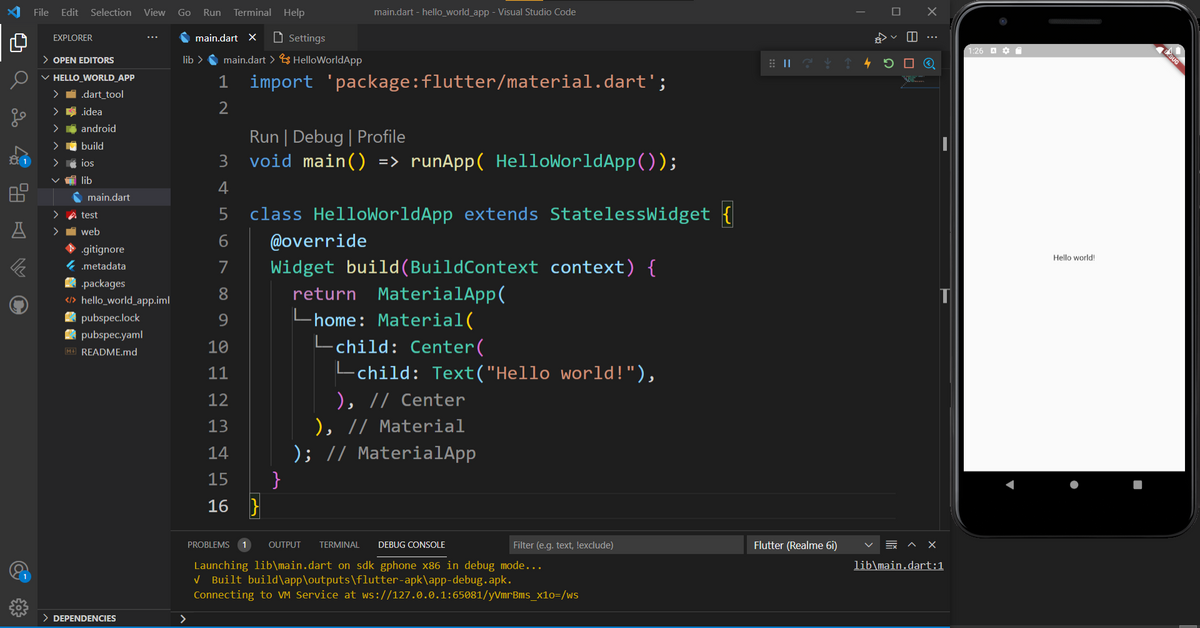
Introduction to Flutter
Flutter is a framework and software development kit (SDK) based on the Dart programming language. SDK stands for “Software Development Kit,” and in the case of Flutter, it refers to a set of tools that assist you in developing, testing, optimizing, and building your cross-platform mobile app. Continue reading to find out more about these two masterpieces (Framework and SDK)!
Flutter was created with the goal of making cross-platform (mobile app) development as simple as possible. The goal is to develop apps for a variety of platforms using a single codebase (like iOS and Android).
Flutter is converted to native machine code for great efficiency, and it comes with a variety of useful tools and features to help you speed up your work.
Flutter vs React Native?
Flutter is all about widgets, which are reusable building pieces that can be used to create attractive user interfaces. Unlike React Native, Flutter does not rely on platform primitives (i.e., the usual UI building blocks provided by Android/ iOS), instead controlling every pixel on the screen. Flutter (and, eventually, you) will have more control over the user interface as a result of this.
Flutter Widgets
Flutter is all about widgets, as previously said.
Widgets are the building pieces that you employ to create your app’s user interface. An example of text output and a button, both would be a widget in flutter.
You can also utilize “invisible” widgets to organize and control other (visible) widgets that you don’t see immediately on the screen. In Flutter, for example, there is a “Column” widget that allows you to arrange additional widgets (such as buttons or text widgets) in a column above each other.
There is no visible drag and drop editor or anything like that to help you create your user interface. Instead, you create a “widget tree,” in which you have one top-level widget (typically the “MaterialApp” widget), which takes additional widgets as inputs, and so on.
In Flutter, you may also create your own widgets; around fact, your entire programme is wrapped in a single core widget that you create. The widgets you create are then made up of other built-in or custom widgets.
Hello World Program in Flutter
This is how your first Flutter ‘Hello World’ app would look:
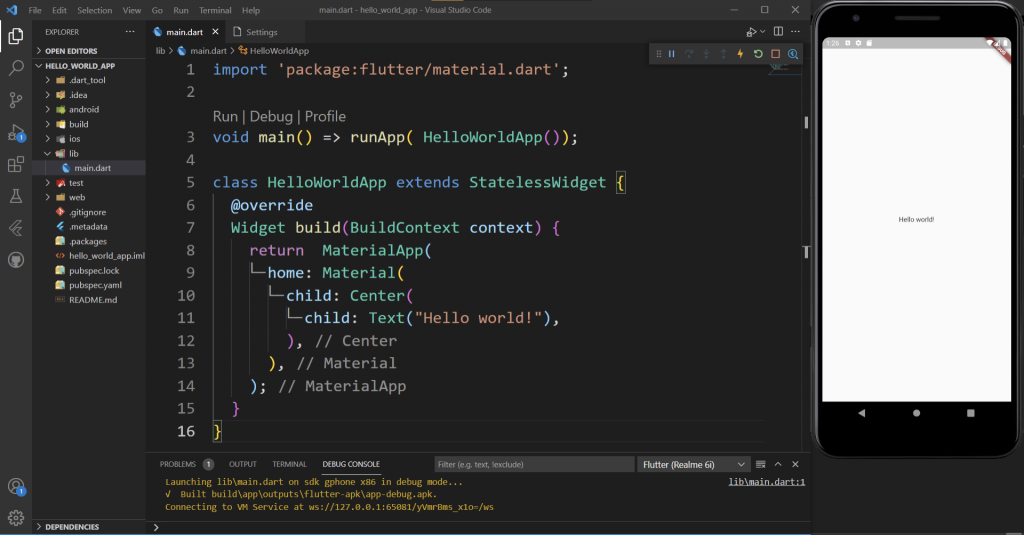
Let’s have a look at this code line by line.
import: 'package:flutter/material.dart';
We’re importing library materials right now. ‘Dart’, which has a large number of material widgets that follow the material design standards.
Widgets are the foundation of Flutter apps, we may use some of the provided widgets by flutter: Buttons, input fields, lists, tables, dialogues, tab bars, card views, and so on are all examples of user interface elements. It’s all up to us!
void main() => runApp(new HelloWorldApp());
The main function is the app’s starting point; it runs the runApp method, which fills the screen with the widget sent in as an argument, which is the root widget.
class HelloWorldApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
}
}
Above is the main widget of our application. The widgets you’ll use to create your app might be stateful or stateless.
Stateful vs Stateless Widget
A stateful widget has a changeable state defining it. The createState() function must be implemented by this type of widget.
A stateless widget, on the other hand, is one that has no internal state, such as an image or a text field. The construct() function must be implemented by this type of widget.
Our widget is stateless, as we’ll just be displaying “Hello world” on the screen the entire time.
return MaterialApp ( home: Material( child: Center( child: Text(“Hello world!”), ), ), );
Additional widgets of our code:
MaterialApp (a material design widget wrapper)
Material (a component of “material”),
Center (a widget that centers objects inside it)
Text (a text field widget).
Each widget includes a collection of characteristics (some required, some optional) that specify the widget’s composition (home, child, children), visual features (decoration, location, style), and behavior (home, child, children) (on click listener). We have complete our Hello World App and its analysis. You’ll get to play with additional widgets as you spend more time working with Flutter, and you’ll come across more use cases where you’ll need to incorporate extra characteristics.