How to Control CSS with jQuery
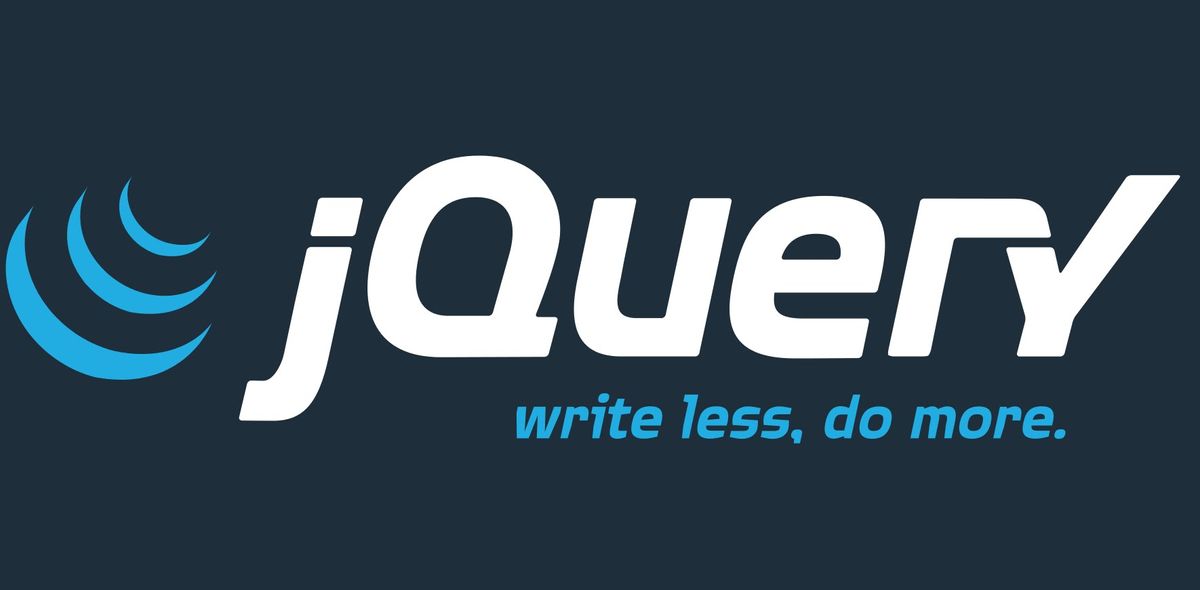
jQuery is a powerful javascript framework. It is fun to control CSS with jQuery, you can easily manage CSS with jQuery, can add/remove css properties, classes and all other cool stuff by jquery Here is a simple guide on how to Control CSS with jQuery.
Add/Remove CSS class with jQuery.
1. Load CSS sheet and jQuery.
2. Give id to the block or paragraph to which you want to add/remove css class
This is the sample text paragraph
3. Use jquery to add CSS class
4. you can also add button to execute jquery, e.g
write query on button click
Add/Remove CSS properties with jQuery
1. add css property
$('#text1').css('color','blue');
2. remove css property
$('#text1').css('color','');
Full HTML Code for Managing CSS with jQuery
Manage CSS with jQuery add css classes with jquery
This is the sample text paragraph
This is how you manage CSS with jQuery