Dockerize Flask Service - Docker and Flask in 5 Easy Steps
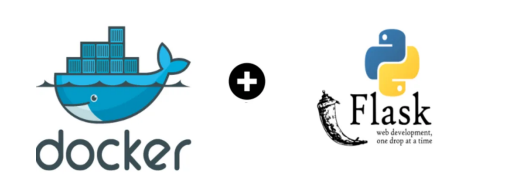
In this tutorial, we will walk through the process of Dockerize FlasK Python service step by step. Docker and flask is an easy example of containerizing a python application.
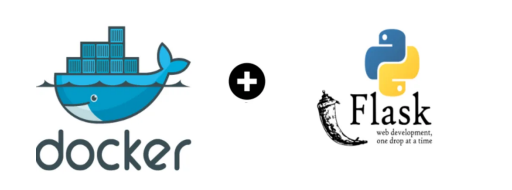
Docker is a platform that offer containerization solution for software applications, and python is our favourite and powerful language. Let’s combine the power of both.
What about Flask, this is a web framework that is written in python and use to build web applications using python.
In current tutorial, we will be using web python application. For very basic or command line python application (hello world program) using docker, please follow this tutorial
1. Prerequisites
Before we begin, ensure that you have the following tools installed on your system:
- Docker: Download and install Docker from the official website.
- Python: Download and install Python from the official website.
2. Create a Python Service
For the purpose of this tutorial, we will create a simple Python service that exposes a “Hello, World!” message via a REST API using the Flask web framework.
Create a directory for your project and create a Python script named app.py
with following code:
# app.py
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0')
Now, create a requirements.txt
file to list the Python packages required for your service, with the following line in it:
Flask==2.0.1
3. Write a Dockerfile
A Dockerfile is a script used to build a Docker image. Create a file named Dockerfile
(no file extension) in the same directory as your Python script app.py and requirements.txt
with the following content:
# Use the official Python image as the base image
FROM python:3.8-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install Python dependencies
RUN pip install --no-cache-dir -r requirements.txt
# Expose port 80
EXPOSE 80
# Define the command to run your application
CMD ["python", "app.py"]
You can name this file: Dockerfile without any extension
This Dockerfile does the following:
- It starts with the official Python 3.8 image.
- Sets the working directory to
/app
in the container. - Copies the contents of the current directory (your Python code and
requirements.txt
) to the container. - Installs the Python dependencies listed in
requirements.txt
. - Exposes port 80 (the default for HTTP).
- Specifies the command to run your application, which is
python app.py
.
4. Build the Docker Image
Navigate to the directory containing your Dockerfile and Python code in your terminal and run the following command to build the Docker image:
docker build -t python-service .
-t python-service
assigns the name “python-service” to your Docker image. You can choose any name you like. This is to tag the docker image with specific name.
5. Run the Docker Container
Now that you have built the Docker image, you can run a Docker container from it. Use the following command:
docker run -d -p 8080:80 python-service
-d
runs the container in detached mode.-p 8080:80
maps port 8080 on your host machine to port 80 in the container.python-service
is the name of the image you built earlier.
Congratulations! You’ve successfully Dockerized a Python service. Your Flask-based “Hello, World!” service is now running in a Docker container and accessible at http://localhost:8080
in your web browser.