Python Regular Expression Easy Tutorial – II
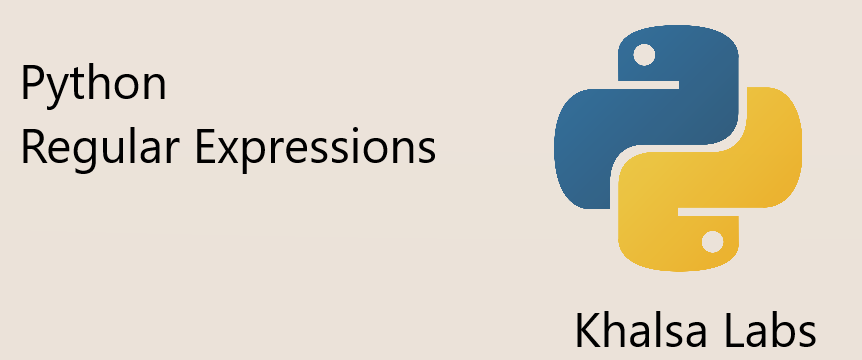
In Lesson 2 of Python Regular Expression Easy Tutorial, we’ll learn how to find text of specific pattern. For example the text patterns like – he99, tintin@email.com , PQ675a, etc
In this Python Regular Expression Easy Tutorial we will step it up to another level. To find these type of strings we have to make the search pattern using some special Characters.
How to Use special Characters in Python:
import re text = "gone123" patt = "[w]" print re.search(patt,text).group()
will match only first occurring word
you can also do this similarly with compile() method in more efficient way:
import re text = "gone123" m = re.compile("[w]") print m.search(text).group()
output will be same as above.
However, re.comile() is used to make object of our pattern, and is used basically in Object Oriented Programming Style.
Some special Characters for pattern matching are:
Keyword Function
w match world
Text = “gone123” Pattern = [w] Result = g
d match decimal (digits)
Text = “gone123” Pattern = [d] Result = 1
s match whitespace
Text = “go to home” Pattern = [w][s][w] Result = o t #will search first occurrence of Letter Space Letter.
n match newline
b match boundary
More Special Operators (also Called Meta characters / WildCards):
+ Matches 1 or more occurrence of preceding character
* Searches for 0 or more occurrence of preceding character
? Matches 0 or 1 character of preceding pattern.
. Matches any one character at its place.
Another pattern = “h.s” will match : has, his, hus
[ ] match range of character ,
e.g [a-z] matches all a to z, [0-9] matches all digits 0 to 9
EXAMPLE IN PYTHON
import re text = "gone 123" m = re.compile("[w]+") print m.search(text).group()
output : gone